Variable Initialization in C++ is a topic directly related to the Assignment operator. The very assignment operator lets you assign the value to an identifier.
int geek = 404; // the assignment operator is denoted by =
Similar to the assignment, we’ve another concept of initialization in C++. In this blog, we’ll learn and get to know about the initialization concept in C++ and how to use them in real-time programming to make your program more accurate and efficient.
The Concept of Initialization
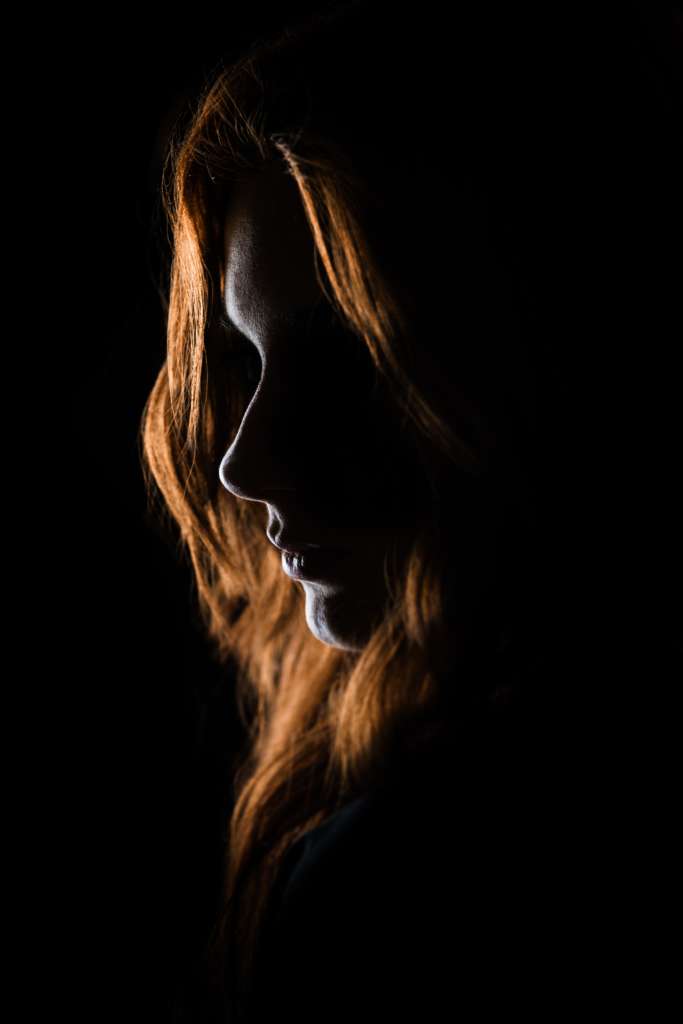
Initialization is the basic concept of originating a variable in C++ in the simplest way possible.
For example, check this code.
int geek; // The identifier geek is initialized
geek = 404; // geek is assigned the value
float pi = 3.14; // Initializing and assigning at the same time
Hence the code above is the core concept behind initialization, i.e., creating a new variable is the whole idea behind initialization. But the topic doesn’t end there. In C++, we have a more prominent ideology to replace the concept of assignment operator while initializing for more accuracy and less ambiguity (ambiguity roughly means confusion for the compiler interrupting other parts of code).
Variable Initialization
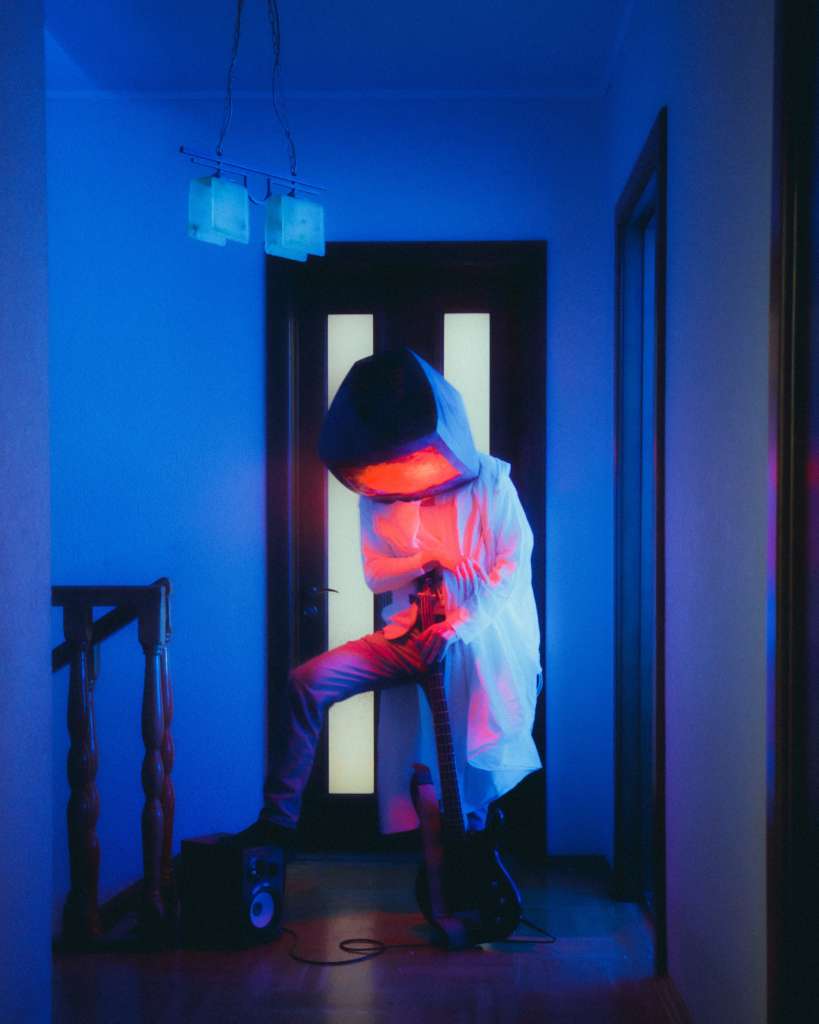
Initialization of Variables always takes place before they are used to store a particular value in the memory. After initialization only, the process of assignment could take place. So, there are two effective ways to initialize the C++ Variables.
- C Style Initialization Syntax
- C++11 List Initialization Syntax
C Style Initialization Syntax
The C Style Initialization Syntax for variable declaration is the most commonly used form of variable declaration. It is straightforward and effortless as this universal variable declaration also occurs in other languages like java.
Syntax
int num = 101; // C Style Initialization of integer number valued at 101
Example
#include <iostream>
using namespace std;
int main(){
//C Style Initialization Syntax
int age = 51;
cout<<"My Dad's age is: "<<age;
return 0;
C++11 Style List Initialization Syntax
The C++11 Style List Initialization Syntax is the method by which most beginners are unaware. Many people don’t know how to use it, why to use it, and why we prefer it over C Style Initialization Syntax.
C++11 Style Initialization Syntax is another, and yet one of the best, ways to create variables as it performs a clean and fresh variable initialization. You’ll understand this part better in the C++11 Syntax vs. C Style Syntax difference section.
Syntax
int num {808}; // C++11 Style Initialization of integer number valued 808
Example
#include <iostream>
using namespace std;
int main(){
//C++11 List Style Initialization Syntax
int age {23};
cout<<"My Dad has experience of more than "<<age<<" years.";
return 0;
}
Variable Initialization: C-Style VS C++11 List Style
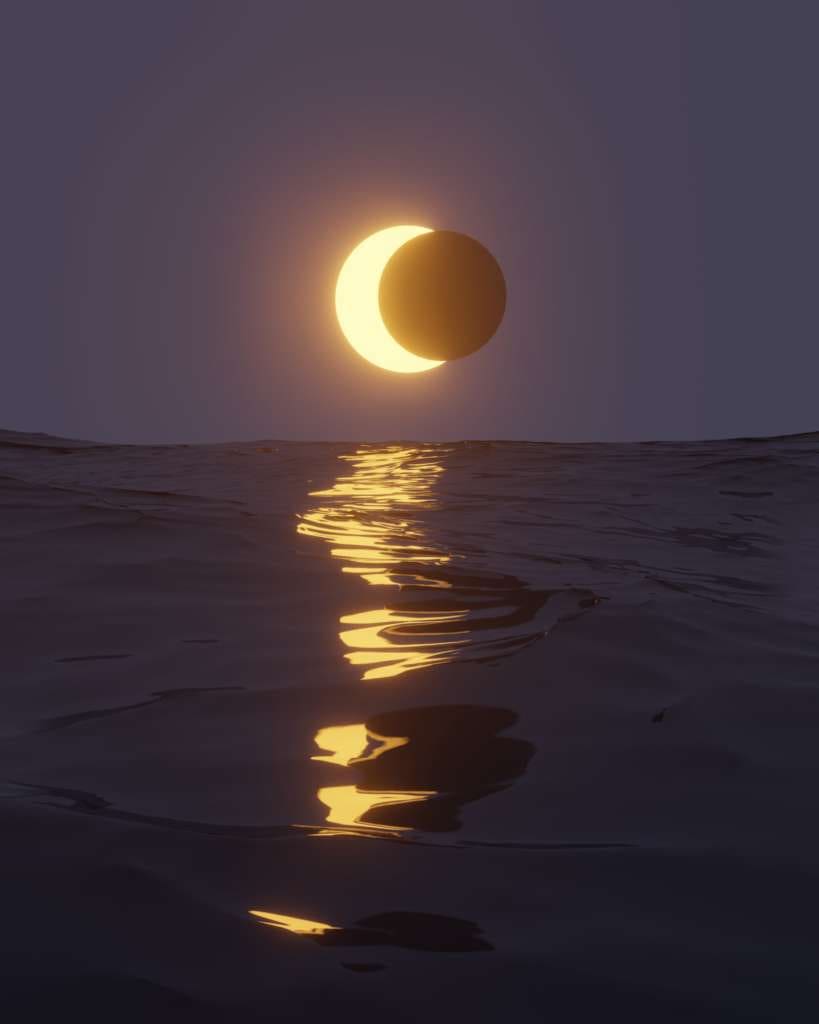
Diving Deeper into C-Style Initialization Syntax
Before concluding, let me take forward the understanding of the concept of initialization to obtain the conclusion with better clarity.
As we already know, the “Initialization of Variables always takes place before they are used to store a particular value in the memory.” In the exact period when the initialization process begins in real-time, the compiler checks the available memory space so it can store the variable’s value in that memory space.
If we break down the C Style initialization properly, we know that the initialization and the assignment are two different processes.
int x; //Initialization
x= 15; //Assignment
int y = 20; //Initialization & Assignment both together
There’s a concern with this default approach.
When we initialize the variable, it does not perform clean initialization. It just links the variable with the memory space in the initialization process.
Let me explain to you with a better practical explanation.
#include <iostream>
using namespace std;
int main(){
// C-Style Initialization Syntax
cout<<"Hellew Geeks!"<<endl; //Typical Greeting
int x; //Initializing the Variable
cout<<x; //Printing the Variable
return 0;
}
You must’ve noticed that we’ve not assigned any value and printed it right after its initialization. Well, that’s the whole thing; we’re here checking the initialization.
Note: Don’t use OnlineGDB and any other compiler to run this code. This code experiment needs a System Compiler to work appropriately. Online compilers are virtual machines with no memory value stored by other programs, so that they won’t work.
OUTPUT
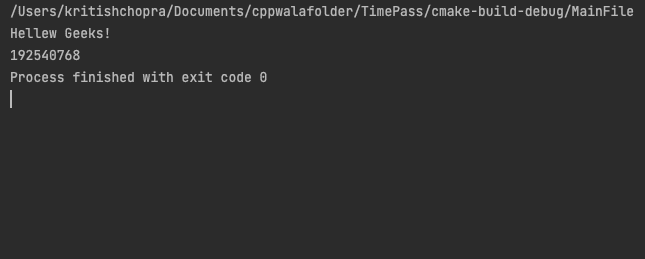
Hellew Geeks!
192540768
Process finished with exit code 0
You’ll be surprised to see the random numbers in the output that we never entered or assigned. These are the numbers stored by other programs in the same memory address our compiler is now using for our variable. In short, our compiler didn’t “CLEANED” the memory address before reusing it.
Furthermore, suppose we dive deeper into this syntax now. In that case, you can quickly realize that when you assign the value using the assignment operator, it simply overwrites your value replacing the old value in the memory address.
You can think of it similarly to eating at an uncleaned table in a restaurant/cafe or staying in an uncleaned hotel room. Eww, that’s gross! I know it is, but this is what a programmer feels while using the old C-type Initialization Syntax.
Diving Deeper into C++11 Style List Initialization Syntax
Whereas if we talk about the new C++11 Style List Initialization Syntax, it’s different and has a modern approach than the C Style initialization approach. It initializes with the clean and easy-to-use approach, which not only makes the variable fresh but also minimizes the chances of errors caused by old and random values. It automatically initializes the clean variable with the value “0”.
int x {15}; //Initialization with the value of 15
int y {}; // Just Initialization gives the value 0
It’s a cleaner approach. Let’s check this code out in the real-time compiler. You can use any compiler to run this code, but again I suggest you prefer System Compilers over online virtuals to get real-time results.
#include <iostream>
using namespace std;
int main() {
//Using C++11 Style Initialization Syntax
cout << "Hellew Smarter Geeks!" << endl; //Typical Greeting
int x{}; //Initializing the Variable
cout << x; //Printing the Variable
return 0;
}
OUTPUT
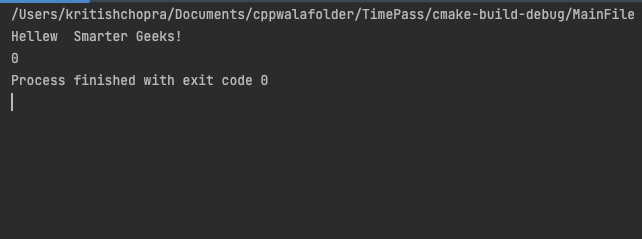
Hellew Smarter Geeks!
0
Process finished with exit code 0
Found the difference?
Hence Satisfied 🙂
So here, you can see that the latest and modern C++11 Style Initialization is far more practical, real-time, and better than the old and outdated C-Style Initialization Syntax. Some Geeks will say that the old C-Style Initialization Syntax is better and easier to use. It’s up to you guys. If you’re finding C-Style easier and more practical, Stick to it! You can use and depend on it if you want to, and it’s pretty good too, but the C++11 Style Initialization is better and more practical and modern as we think and industries know about it.
Conclusion
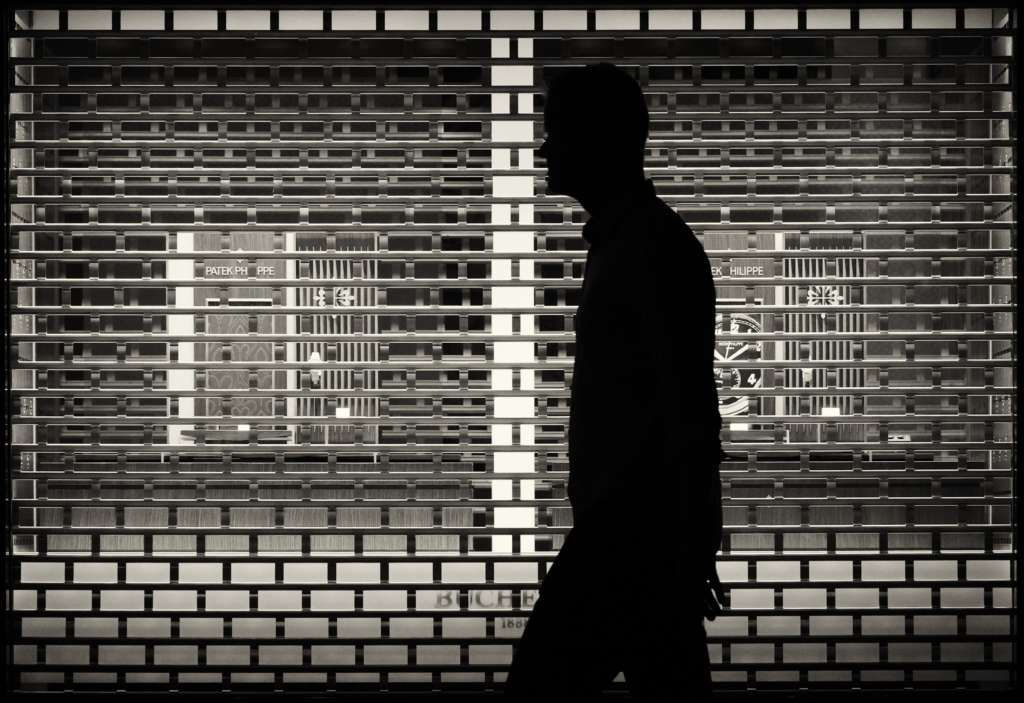
In this blog, we learned about initialization and the old and new method to perform it, the ways, the difference, the whole ideology, mindset, mechanism, and advantages, as well as the drawbacks. The market and people’s habit is also what you’ve studied, and you also got to know about the basics and how things have changed over time. It’s all not over yet! GeekonPeak’s full of treasures of knowledge. To access the Knowledge chest, There’s something you must do!
Do subscribe to our almighty newsletters to stay updated and geeky and to receive the techy tips directly in your inbox, specially researched and implemented by geeks just for you. You can also like our blog without logging in just show us your love by pressing the heart icon at the end of the blog. You can also show your response and love in the comment section below. Again, you don’t have to log in, so it’s easy-peasy lemon squeezy. Suggestions and improvements are always welcome.
You can also show us your love on social media, i.e., Instagram, Facebook, Twitter, and Linkedin, and you shall also check out our new blogs regularly. We’re also trying hard to provide the best content by using premium tools, and we’re expecting you to share our website using word of mouth if you’re finding our content worth sharing, you can also share using social media, and it’ll truly help us a lot. We’re counting on you for the support of our website, and stay geeky until next time.
2 Comments
felomim
Do HTML + CSS
CINORAL
Do HTML + CSS + JS