Conditional Statements in C++, aka C++ if else statements, is a stage in learning programming where you’re putting another significant step in learning programming. If you’ve studied programming from the beginning till here, that means you’ve already gone through the core basics and syntax of C++. You’ll learn to program and use your brain logically from this step. You’ll learn to handle the code’s control flow and control the code using C++ if else statements.
This is the best and the most detailed blog written by the author,i.e., “Kritish” (me), to date. This could also be the best C++ if else blog on the web. So please read this very C++ if else – Complete guide blog carefully and like (heart button on the bottom) and comment about the blog on our website.
C++ If statements
if statement is a block of code used to control the flow of the program. Using the if statement, we can control the flow by evaluating whether the specific condition is met.
the if statement code block only gets executed when the specific condition is met; otherwise, the lines of code inside the if code block will be skipped, and the execution will be continued.
Diagram
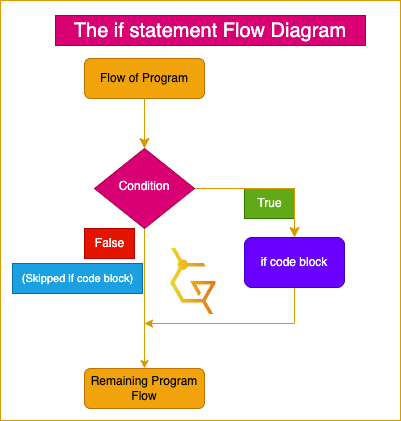
Using the diagram above, we can quickly evaluate the following:
The flow of the program is executed continuously until the conditional statement arrives.
The condition evaluates where it is true/false and decides its path to go inside the if code block and execute it or skip the if block and continue the remaining execution.
If the condition evaluates to true, the code inside the if code block gets executed.
And if the condition turns out to be false, then the code inside the if code block gets skipped, simple.
Syntax
if (condition){
//This is the if block.
//The code inside if block will only be executed...
//...when the condition becomes true.
}
Things to Remember in C++ If Syntax
The syntax is pretty simple:
The if (condition) requires a valid condition, resulting in a boolean (0 = false/1 = true) value, as it will help the condition to make the appropriate decision accordingly.
Based on the if (condition), it will return a boolean value and decide where to go.
Providing (condition) is necessary for the if block.
Scenario
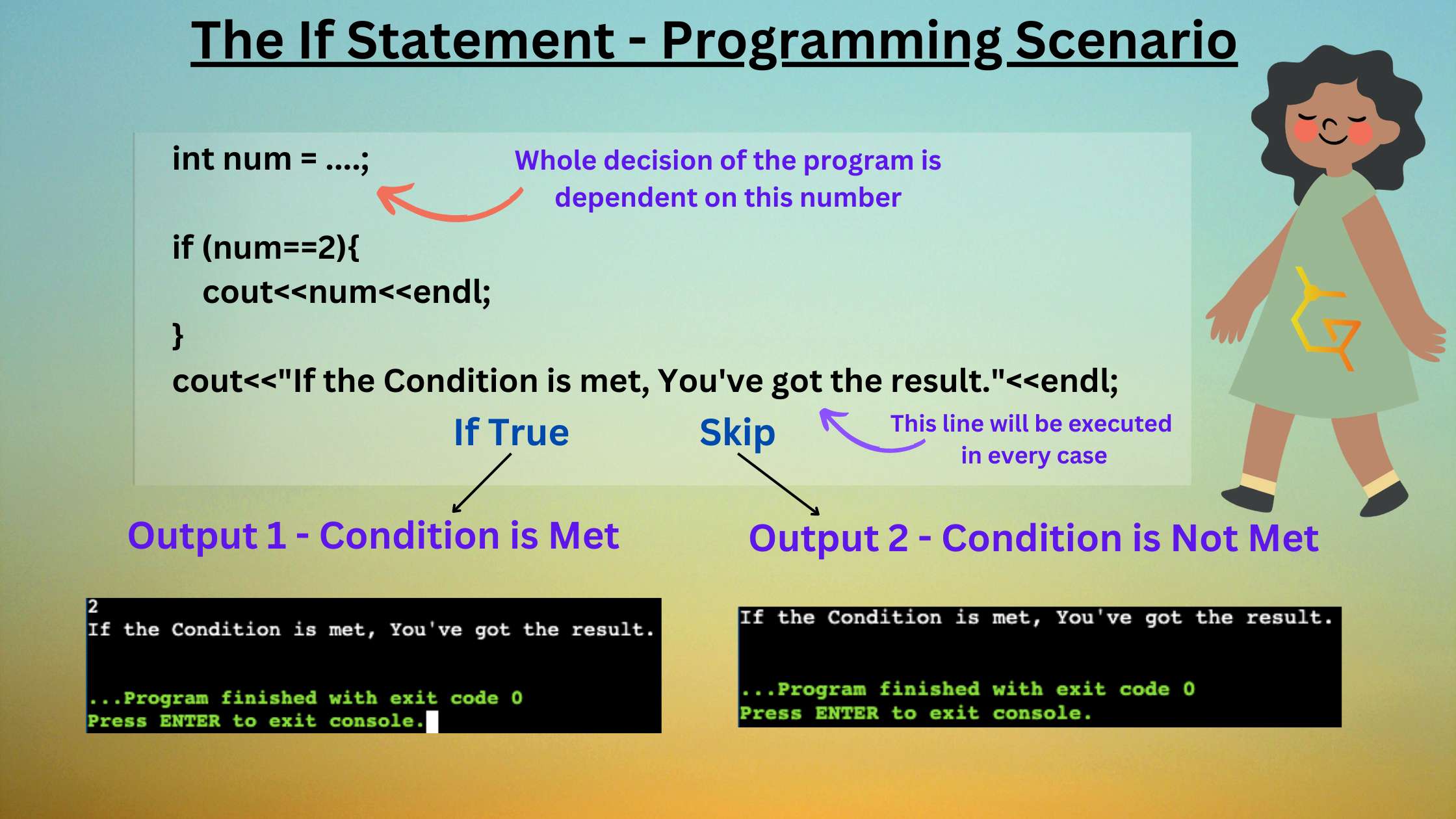
Using the Scenario above, we can evaluate the following:
Firstly, it is easy to interpret that this code prints the number 2 inside the if statement if it gets inside the conditional block.
The entire decision is upon the user’s input; if input meets the condition, the number gets executed (the output 1 above); otherwise, it skips the if code (the output 2 above).
After ending the conditional statements, the line will execute every time as it is the remaining program flow.
Example
#include <iostream>
using namespace std;
int main(){
bool good;
cout<<"Are You Good (0/1): "; // (0 = false, 1 = true)
cin>>good;
cout<<"I am a";
if (good==true){
cout<<" good";
}
cout<<" geek";
return 0;
}
Output 1: The Condition is Met With the If Statement
Are You Good (0/1): 1
I am a good geek
Output 2: The Condition is Not Met With the If statement
Are You Good (0/1): 0
I am a geek
Explanation
This code is straightforward to interpret. In the above code, a simple boolean variable is created, and the user is asked to input whether they are good or not in the boolean value (0=False, 1=True).
If the user enters he is not good (0=false), then the code will not enter the if block, and then the user will receive the output as I am a geek, as shown in output 2.
If the user entered that he’s good (1=true), then the code inside the if statement evaluates the good into the statement, giving the output I am a good geek, as shown in output 1.
C++ If else statements
C++ if else statement is the most used and logical method to control the flow of the program in C++. Using the C++ if else statement, we can control the flow of the program more efficiently, and the program will make more logic.
In this statement, nothing is skipped either the program flow enters in the if block or it enters in the else block; hence there is no escape; it has to go through one of them.
Diagram
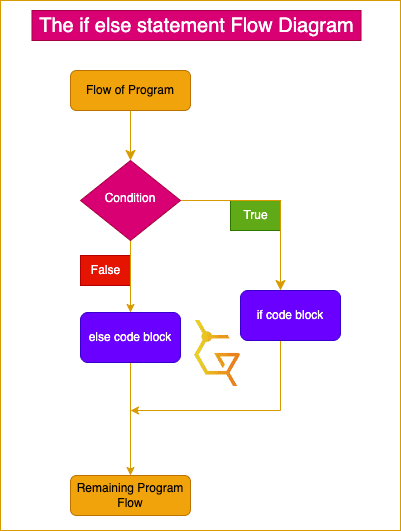
Using the diagram above, we can quickly evaluate the following:
The flow of the program is executed continuously until the conditional statement arrives.
The condition evaluates where it is true/false and decides its path to go inside the if code block and execute it or to get into the else block for execution. Then after ending the conditional C++ if else statement, it continues the remaining execution.
If the condition evaluates to true, the code inside the if code block gets executed.
And If the condition evaluates to false, the code inside the else code block gets executed.
Syntax
if (condition){
//If the condition is met...
//...if block will be executed
}
else {
//Otherwise this (else) block will be executed...
//...Nothing will be skipped
}
Things To Remember in C++ if Else Syntax
The syntax is again pretty simple:
We know Providing (condition) is necessary for the if block, but we never provide a condition to the else block. According to the program’s logic, one of both conditions (if/else block) must be executed in the program.
Scenario
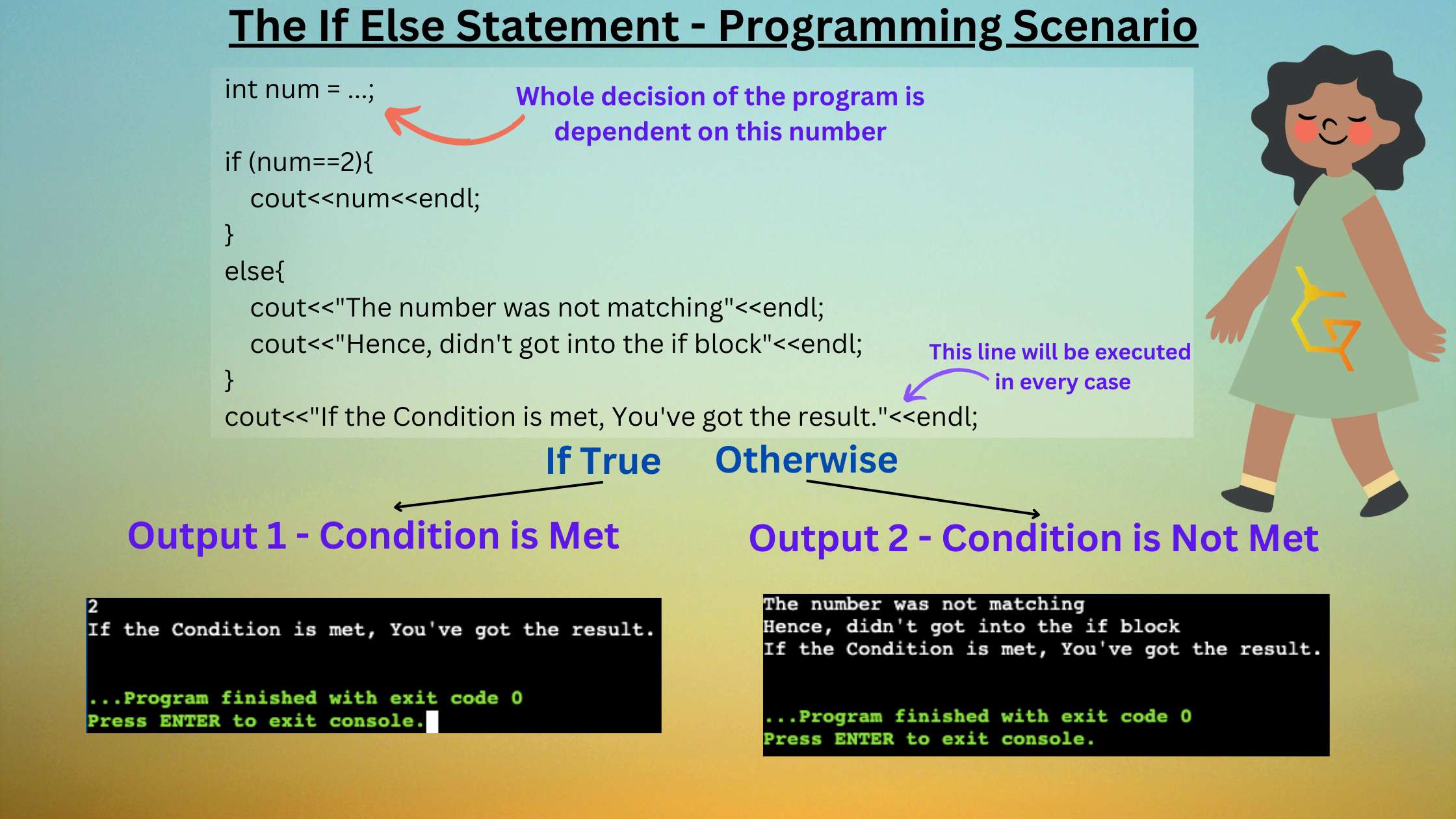
Using the Scenario above, we can evaluate the following:
Firstly, it is easy to interpret that this code prints the number 2 inside the if statement if it gets inside the conditional block.
If the program flow failed to get into the if block of code., the else block will be executed, i.e., “The number was not matching.”
Another line is being printed in the else block, clarifying the code didn’t go into the if block.
The entire decision is upon the user’s input; if input meets the condition, the code in the if block gets executed (the output 1 above); otherwise, the code in the else block gets executed (the output 2 above).
After ending the C++ if else conditional statements, the line will execute every time as it is the remaining program flow.
Example
#include <iostream>
using namespace std;
int main(){
bool good;
cout<<"Are You Good (0/1): "; // (0 = false, 1 = true)
cin>>good;
cout<<"I am a";
if (good==true){
cout<<" good";
}
else{
cout<<" bad";
}
cout<<" geek";
return 0;
}
Output 1: The Condition is Met With the If Statement
Are You Good (0/1): 1
I am a good geek
Output 2: The Condition is Not Met With the If statement, Therefore else statement
Are You Good (0/1): 0
I am a bad geek
Explanation
This code is, again, straightforward to interpret. In the above code, a simple boolean variable is created, and the user is asked to input whether they are good or not in the boolean value (0=False, 1=True).
If the user enters he is not good (0=false), then the code will enter the else block, and then the user will receive the output as I am a bad geek, as shown in output 2.
If the user entered that he’s good (1=true), then the code inside the if statement evaluates the good into the statement, giving the output I am a good geek, as shown in output 1.
If, else-if, else statements
What if we’ve more than one statement to continue the control flow, we mustn’t use multiple if conditions as the following statement to control a single program flow. Then what’s the big idea?
We often have to check more statements to continue the program flow before entering the else block. And we do this by using else if blocks. The If, else if, else is a complete trilogy of control flow statement blocks that you’ll ever need to control almost any control flow in any programming language. Let’s check how.
Diagram
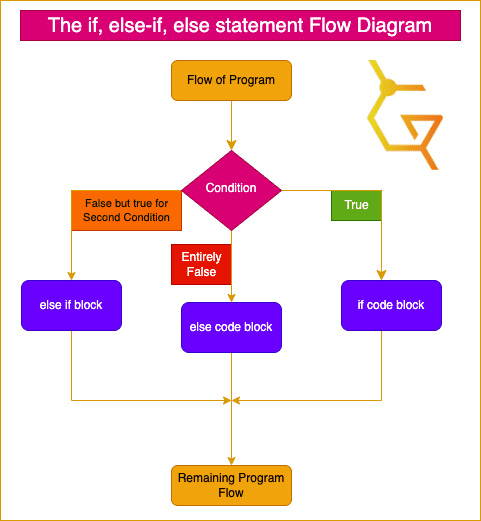
Using the diagram above, we can quickly evaluate the following:
The flow of the program is quite different from the standard C++ if else statement approach.
In this method, there are 3 parameters for a condition to check.
Firstly, the program will evaluate the true/false from the condition and decide whether it has to go inside the if code.
In the case it’s not suitable with the condition to go inside the if block, it will again evaluate whether it can get into the else if block, as it also requires a condition to check.
And if finally, the program is not suited to go into both if block and else if block. Then the program will finally enter the else block as it’s the last choice.
Therefore these are the 3 paths where a program shall continue the remaining execution.
There can be any number of else if conditions between a single if and else block of code.
Syntax
if (condition){
//If the condition is met...
//...if block will be executed
}
else if(condition){
//If the first condition is not met...
//...and the second (this) condition is met...
//...This block of code will be executed
}
else {
//Otherwise this block will be executed...
//...Nothing will be skipped
}
Things To Remember in C++ if, Else if, Else Syntax
In the case of else if also, providing (condition) is necessary just like the if block, but we again never provide a condition to the else block. According to the program’s logic, one of the matching conditions must be executed in the program, and at the end, if none of the conditions matches, the else block will be executed.
Scenario
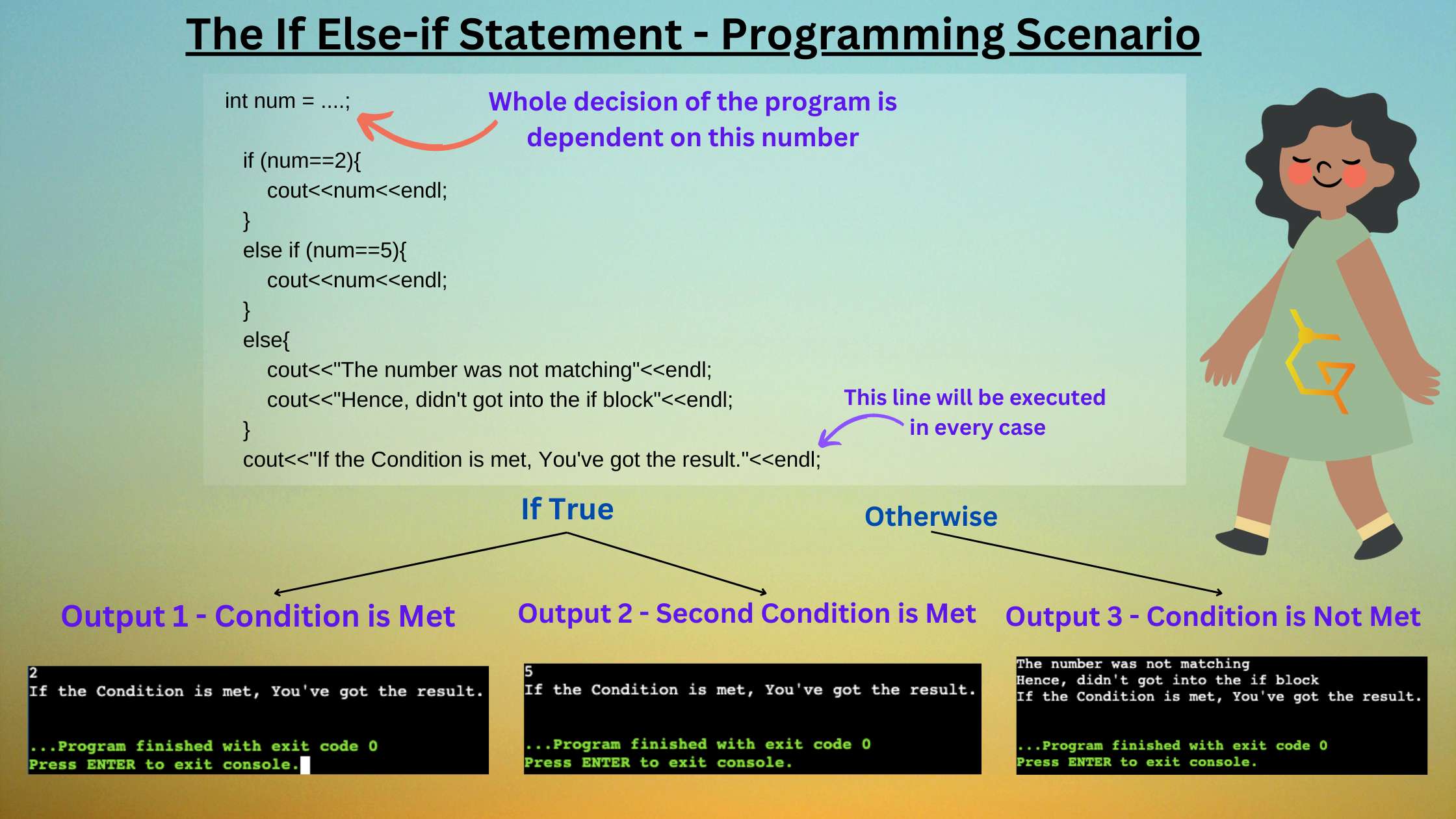
Using the Scenario above, we can evaluate the following:
Firstly, it is easy to interpret that this code prints the number 2 inside the if statement if it gets inside the conditional block.
Then an else if condition is also used to check whether the condition matches or not before the else block; in our case, it is checking whether the user input is 5.
If the condition matches and the input is 5, the else if block gets executed that prints the number 5 and continues the remaining program flow.
Otherwise, the else block will be executed, i.e., “The number was not matching”, if the program flow failed to get into the if block of code.
Another line is being printed in the else block, clarifying the code didn’t go into the if block.
The entire decision is upon the user’s input; if input meets the condition, the code in the if block gets executed (the output 1 above), or the code in the else if block gets executed (the output 2 above); otherwise, the code in the else block gets executed (the output 2 above).
After ending the C++ if else conditional statements, the line will execute every time as it is the remaining program flow.
Example
#include <iostream>
using namespace std;
int main(){
int hour;
cout<<"Input current hour (24 hour format): ";
cin>>hour;
if (hour>=0 && hour<4){
cout<<"Mid-night Time";
}
else if (hour>=4 && hour<12){
cout<<"Morning Time";
}
else if (hour>=12 && hour<16){
cout<<"Noon Time";
}
else if (hour>=16 && hour<20){
cout<<"Evening Time";
}
else if (hour>=20 && hour<=24){
cout<<"Night Time";
}
else{
cout<<"Invalid Time Input";
}
return 0;
}
Output 1: The Condition is Met With the If Statement
Input current hour (24 hour format): 3
Mid-night Time
Output 2: Random Condition is Met With Else-If statement, But not if statement
Input current hour (24 hour format): 21
Night Time
Output 3: The Condition is Not Met With both statements, Therefore else statement
Input current hour (24 hour format): 28
Invalid Time Input
Explanation
In the above example, firstly, an integer variable is initialized, and user input is stored in the variable; pretty straightforward.
Then based on the user input, various conditions will be checked, and the final decision will be made. Let’s understand this in order.
Firstly if the time is between 0 and 4, i.e., including 0 and excluding 4, it will go into the if condition, which will return “Mid-night Time.”
If the time is between 4 and 12, i.e., including 4 and excluding 12, it will go into the else if condition, which will return “Morning Time.”
If the time is between 12 and 16, i.e., including 12 and excluding 16, it will go into the else if condition, which will return “Noon Time.”
If the time is between 16 and 20, i.e., including 16 and excluding 20, it will go into the else if condition, which will return “Evening Time.”
If the time is between 20 and 24, i.e., including 20 and also including 24, it will go into the else if condition, which will return “Night Time.”
Finally, if the time is not between 0 and 24 (all including), the code will enter the else block, returning “Invalid Time Input.”
This is how the example works; it was also easy to interpret and understand, considering your super basics are clear.
NESTED C++ If Else statements
We’ve already studied all the main conditional C++ if else blocks. But what if we want the conditional C++ if else block inside another conditional block? Like if, I’ve put a condition where a student obtains marks between 40 and 100, and again another condition where a student obtains marks between 90 and 100 receives an A+ grade.
In this situation, the first conditional C++ if else block checking between 40 and 100 confirms that the student doesn’t fail and he passes with a valid score.
And another condition confirms that he gets an A+ grade, as he passes with a score between 90 and 100.
This can be done with nested conditional statements, aka nested C++ if else only. Let’s find out how it actually works.
Diagram
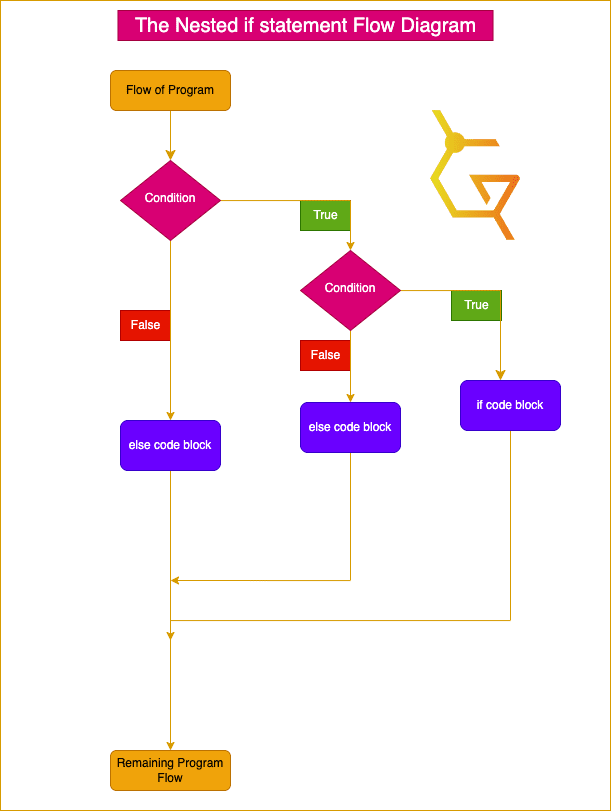
Using the diagram above, we can quickly evaluate the following:
The flow of the program is totally different from all other approaches.
In this method, the nested statement can be inside of any 3 if, else if, else blocks. But currently, we’re assuming we’ve nested inside the if block.
Firstly, the program will evaluate the true/false from the condition and decide whether to go inside the if code or else block.
If the program enters the if block, again, it faces 2 paths to take, and it will take the one satisfying the condition of the program.
And the execution of the nested statement begins and ends after the whole conditional statement to continue the remaining program flow.
And if the program doesn’t enter inside the outer if block, as usual, it will enter the outer else block, and the remaining execution will continue after the execution of the else block.
Syntax
if (condition){
//When we enter the if block and the condition is met...
//...then again some conditions shall be checked
if (condition){
//If the inner condition is met...
//...if block will be executed
}
else if(condition){
//If the first inner condition is not met...
//...and the second (this) inner condition is met...
//...This block of code will be executed
}
else {
//Otherwise this inner block will be executed...
//...Nothing will be skipped
}
}
else{
//if the outer if condition is not met...
//then else block will be executed
}
Things To Remember in Nested C++ If Else Syntax
In the case of nested if, everything is the same. The same if, else-if, else blocks.
The indentation takes the blocks inside and makes it nested, and the braces {} decide the boundary of the blocks of code.
You can nest all 3 if, else-if, else blocks in any of the 3 if, else-if, else blocks. That means you can nest any block anywhere until and unless it follows the syntax and is in order.
The Program ~ Nested C++ If Else
Program 1: Write a program using nested if statements to calculate the appropriate grade from the marks.
#include <iostream>
using namespace std;
int main(){
//Part 1: Initialization & Input
int marks;
cout<<"Enter Marks: ";
cin>>marks;
//Part 2: The Main Game
//Part 2, Phase 1: Checking the Input Value
if (marks>100 || marks<0){
cout<<"Retry with a Correct Value";
}
//Part 2, Phase 2: Grading with Appropriate Score
else if (marks>=40){
if (marks>=90){
cout<<"You've Been Graded with A+, Congrats!"<<endl;
}
else if (marks>=80 && marks<90){
cout<<"You've Been Graded with A, Congrats!"<<endl;
}
else if (marks>=70 && marks<80){
cout<<"You've Been Graded with B, Improve!"<<endl;
}
else if (marks>=60 && marks<70){
cout<<"You've Been Graded with C, Improve!"<<endl;
}
else if (marks>=50 && marks<60){
cout<<"You've Been Graded with D, Poor!"<<endl;
}
else{
cout<<"You've Been Graded with E, Very Poor!"<<endl;
}
}
//Part 3, Phase 3: The Failure
else{
cout<<"You've Failed!"<<endl;
}
return 0;
}
Output 1: The Condition is Met With the If Statement
Enter Marks: 108
Retry with a Correct Value
Output 2: The Condition is Met With the random Nested Statement
Enter Marks: 95
You've Been Graded with A+, Congrats!
Output 3: The Condition is Not Met With Any Statement
Enter Marks: 32
You've Failed!
Explanation
This program is the straightforward and practical implementation of the nested C++ if else statements. By understanding this, you’ll gain clarity of the nested C++ if else topic.
In the first part, we’ve started with initializing an integer variable, “marks,” taking user input for marks and storing it.
//Part 1: Initialization & Input
int marks;
cout<<"Enter Marks: ";
cin>>marks;
In the second part, we’ve to understand the main logic; that’s why it’s “the main game.”
Part 2, Phase 1 confirms whether the marks are between 0-100. Hence it checks and validates whether the marks entered by the user are correct or not. Therefore technically, the marks entered by the user should not be less than 0 and more than 100. It will return “Retry with a Correct Value.”
//Part 2, Phase 1: Checking the Input Value
if (marks>100 || marks<0){
cout<<"Retry with a Correct Value";
}
Part 2, Phase 2, is where we get our main score in the program. Let’s understand it in more detail.
Firstly, the else if block checks whether the marks are greater than 40; therefore, if the student passes by getting more than 40, it enters into the else if block.
Inside the else if block, the nested conditional blocks are there to give the appropriate grading.
If a student earns 90 or more marks, he receives an A+, as mentioned in the nested if block.
If a student earns 80 or more marks up to 90 but excluding 90 (therefore up to 89), he receives an A, as mentioned in the nested else if block.
If a student earns 70 or more marks up to 80 but excluding 80 (therefore up to 79), he receives a B, as mentioned in the nested else if block.
If a student earns 60 or more marks up to 70 but excluding 70 (therefore up to 69), he receives a C, as mentioned in the nested else if block.
If a student earns 50 or more marks up to 60 but excluding 60 (therefore up to 59), he receives a D, as mentioned in the nested else if block.
If a student earns failed to earn 50 or more marks, he will receive an E, as he gets it through the nested else block.
//Part 2, Phase 2: Grading with Appropriate Score
else if (marks>=40){
if (marks>=90){
cout<<"You've Been Graded with A+, Congrats!"<<endl;
}
else if (marks>=80 && marks<90){
cout<<"You've Been Graded with A, Congrats!"<<endl;
}
else if (marks>=70 && marks<80){
cout<<"You've Been Graded with B, Improve!"<<endl;
}
else if (marks>=60 && marks<70){
cout<<"You've Been Graded with C, Improve!"<<endl;
}
else if (marks>=50 && marks<60){
cout<<"You've Been Graded with D, Poor!"<<endl;
}
else{
cout<<"You've Been Graded with E, Very Poor!"<<endl;
}
}
Proceeding towards the final phase, i.e., Phase 2, Part 3, if a student has failed to obtain less than 40 marks, he will be failed through the outer else block, hence it will not enter the else if block where the nested statements exist, instead it will go into the else block and get failed.
//Part 3, Phase 3: The Failure
else{
cout<<"You've Failed!"<<endl;
}
return 0;
}
This is how we can solve the problem using nested C++ if else statements, moving towards the following C++ if else program to give you more clarity.
The Program ~ Simple C++ If Else
Program 2: Write a program to detect the number entered by the user, whether odd or even.
#include <iostream>
using namespace std;
int main(){
//Part 1: Creating & Entering Input
int num;
cout<<"Enter Number: ";
cin>>num;
//Part 2: Decision Making
if (num%2==0){
cout<<num<<" is even";
}
else{
cout<<num<<" is odd";
}
return 0;
}
Output 1: The Condition is Met With the If Statement
Enter Number: 8
8 is even
Output 2: The Condition is not Met With the If Statement
Enter Number: 25
25 is odd
Explanation
This program is pretty simple if you’ll get the main logic, as it uses C++ if else statements.
In the first part, an integer variable num is initialized, and user input is taken and stored in the num.
//Part 1: Creating & Entering Input
int num;
cout<<"Enter Number: ";
cin>>num;
The second part is crucial, as the primary decision-making is being done. Let’s understand the logic first.
We know any number which gets divided by 2 is an even number.
Hence, any number divided by 2 will give the remainder as 0, as it’ll be completely divisible.
Therefore we’ve put the condition of any number completely divisible by 2 or returning the remainder as 0 is an even number.
if (num%2==0){
cout<<num<<" is even";
}
Otherwise, it’s an odd number; that’s it
else{
cout<<num<<" is odd";
}
We can solve the problem using the C++ if else statements. This is the end of the program and this jumbo blog on C++ if else statements.
Conclusion
This blog has taught us about C++ if else statements in detail with practical exposure. From this blog, you must learn how every C++ if else statement execute in real time. In the following blog, we shall learn about the switch statement and short-hand if-else statements.
This was the most detailed blog ever, and it took a lot and a lot of money & effort to upgrade the blog’s quality to this level. But the best part is we’ve not done yet! We’ve just begun. We’ve constantly improved the quality to enhance your experience, which shall require your support, and we expect it from you.
We shall start accepting donations soon as we’re spending heavily on the maintenance and the super high-quality content creation & research of the website. You can now support us through the like button on the bottom, sharing this blog, disabling ad blockers, commenting on your thoughts, and connecting with us on socials as well. You may also subscribe to our newsletters and check out our latest blogs from other niches. Perfect Goodbye for now!