The looping is indeed important; by now, you must be aware of this fact, and here we’ll learn about our third and final loop of C++,i.e., the Do-While loop. This is a special type of loop in C++ which is quite different from the other two loops; we’ll learn this fact more effectively while we dive deeper into the topic. But in the beginning, let me take through the basics of the looping concept.
- Looping means repeating a block of code until the condition is satisfied.
- Looping is also a part of control flow statements and conditional statements.
- In the conditional statements, we enter the block which satisfies the condition.
- In loops, the code enters the loop block and executes the line of codes repeatedly when the existing condition is already true with the loop condition, then exit the block when the condition is satisfied (turns false).
- There are mainly three types of loops – for loop, while loop, and do while loop.
- This blog mainly focuses on one significant type of loop, i.e., do-while loops; hence, let’s dive more in-depth by understanding what exactly these do-while loops are and how they work.
This is one of the best and the most detailed blog written by the author,i.e., “Kritish” (me), to date. This could also be the best C++ do-while loop blog on the web. So please read this C++ for loop blog carefully and like (heart button on the bottom) and comment about the blog on our website.
Do While Loops
Do-While Statement is one of the major loops in C++ programming, which executes the loop block at least once before checking the loop conditions. If you want to execute the loop body at least once, even if the loop condition is false (satisfied), Do-While Loop is the method you must use.
Here we’re taking the help of the do block and while block, where we’re using the do block as a loop block. Inside the do block, we code the loop body, and at the end of the do block, we place ours while condition, followed by the semicolon. Therefore do body executes until the program flow reaches the while condition and afterward when it decides to continue to loop or terminate it.
As the do-while loop is super similar to the while loop, we manually create and update the loop variable or iterator.
IMPORTANT NOTE: In this blog, some terms are used, like the condition is satisfied and the condition is not. We want to provide some extra clarity on that for better understanding.
- If the condition is not satisfied, that means the condition is returning true with the situation, or the program flow is turning out to be true with the condition, which means the program flow will enter inside the code block to iterate through the loops statement and satisfy the condition again and again until the condition turns out to be false.
- Suppose the condition turns out to be satisfied. In that case, it means the condition is returning false with the situation, or the program flow is turning out to be false with the condition, which means the program flow will not enter the code block as the condition is already satisfied; therefore, it will skip the loop and continue the remaining program flow.
Diagram
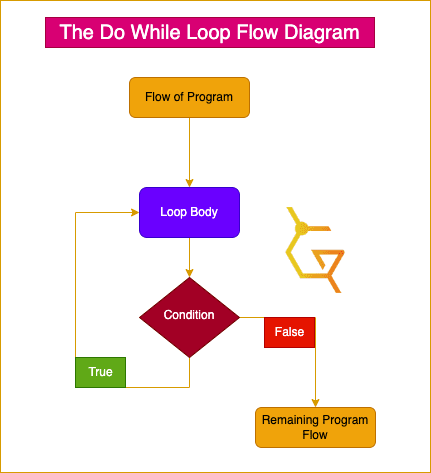
Explanation
Let’s understand the working of the Do-While Loop using the diagram:
- Beginning with the flow of the program, the manual iterator is created in the very first step. As it is very similar to a while loop, we’ve also had to create an iterator manually.
- Then we’ll directly get into the loop body without checking the condition. We’ll put the code in the do block, which will execute the code in the very first step.
- After the iterator updation, which is part of the loop body, the condition is checked, and the loop condition decides whether the condition is satisfied or not.
- If the condition is not satisfied (the condition is still true as per the program flow), then the do block will be looped until the satisfaction of the code (the condition turns out to be false).
- Otherwise, if the condition turns out to be false, then the remaining program flow will be executed.
- The special feature of this loop is the loop block will be executed at least once, even if the loop condition is satisfied (already true).
int iterator = 1;
do {
//loop code block to be executed
iterator++; //iterator update
} while (condition);
C++Explanation
Let’s interpret the super-simple and basic syntax.
- Firstly, the iterator variable is initialized to 1. It is up to the user; you can initialize it to 0 or any number you want.
- Then, we’ll code the loop body using the do block and update the iterator.
- After coding the loop body in the do block, we’ll call the while loop followed by the semicolon. While the loop will contain the condition specified by the user.
- This method will execute the condition at least once before the loop condition check.
Example
#include <iostream>
using namespace std;
int main(){
int times;
cout<<"Enter Times: ";
cin>>times;
int i=1;
do{
cout<<"Geeky Time!"<<endl;
i++;
} while(i<=times);
return 0;
}
C++Output
Case: 4 Times
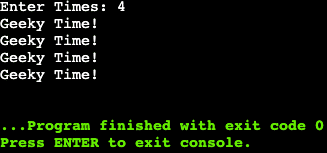
Case: 0 Times
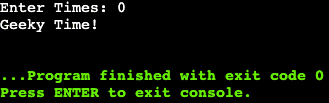
Explanation
The Program is super-simple and easy to understand:
- Firstly we created the ‘times‘ variable and stored the user input.
- Then we created an iterator ‘i’ to 1.
- Then we initialized the do-while loop, where inside the do block, we printed “Geeky Times!” with end line statement. And we also incremented/updated the iterator inside the do block.
- After the do block ends, the while loop checks and decides the program flow. The code has to run until it is less than equals the ‘times‘ variable (user-input), which will print the “Geeky Times!” until the user input exactly.
- But the catch here is, in case 1; everything was perfect. In case 2, we entered zero times, and still, it was printed a single time. This proves the working of a do-while loop, which states the loop block will be executed at least a single time, even if the condition is already satisfied (returning false).
While Loop Versus Do-While Loop
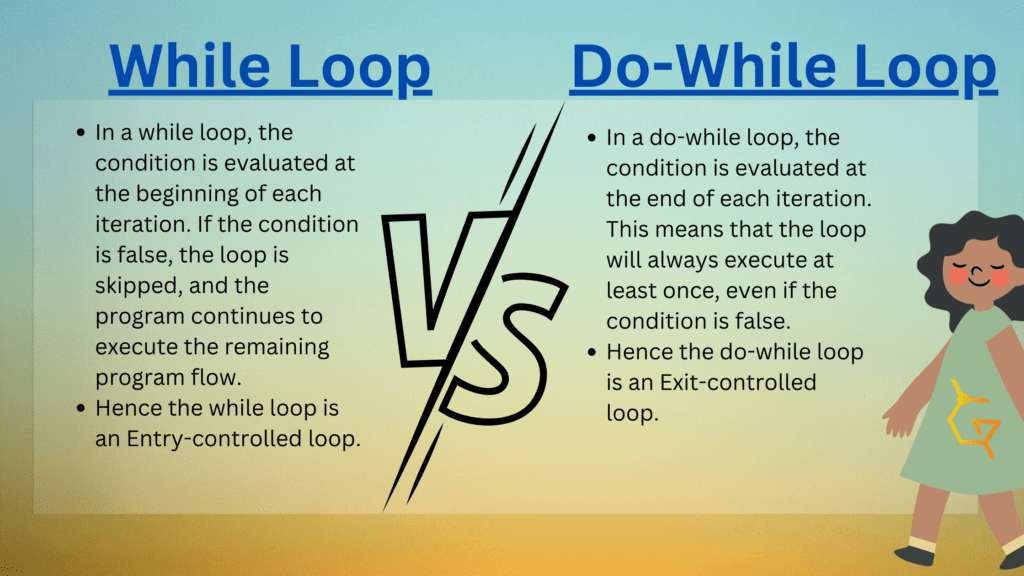
Before diving deeper, let’s understand the basic difference between the while and do-while loop.
The major difference between a while and a do-while loop is the position of the loop and the loop execution.
As in the while loop, the loop condition is placed on the top of the body; that’s why the condition is evaluated before entering the loop body, making the while loop an entry-controlled loop.
Whereas in the do-while loop, the loop condition is placed on the bottom of the body, which evaluates the execution of the loop at least a single time, making the do-while loop an exit-controlled loop.
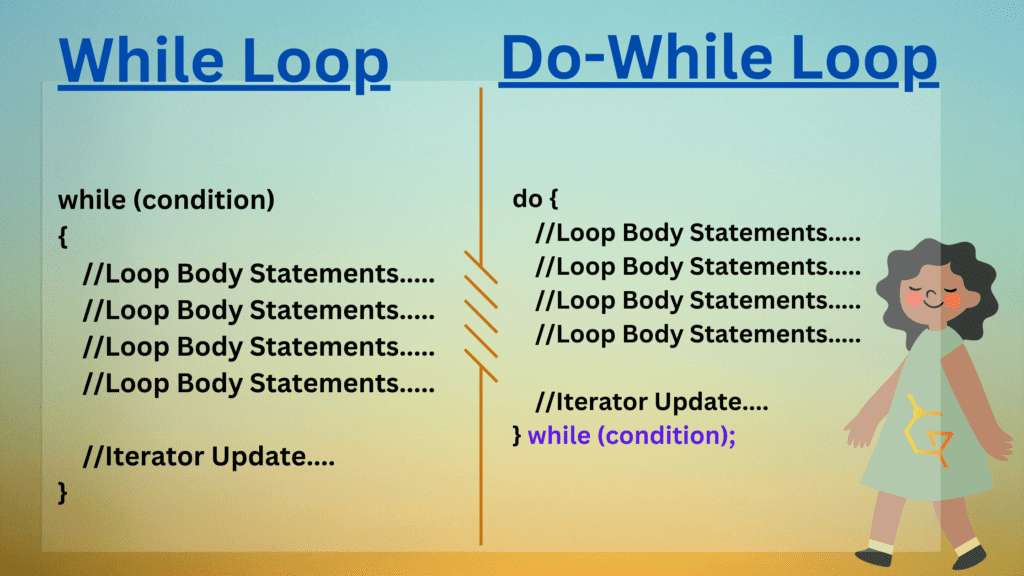
Here is the syntax of both loops for better understanding and reference. We also have an exclusive example for you guys who’ve reached till here. This example will help you to give a clear picture of the difference between the both.
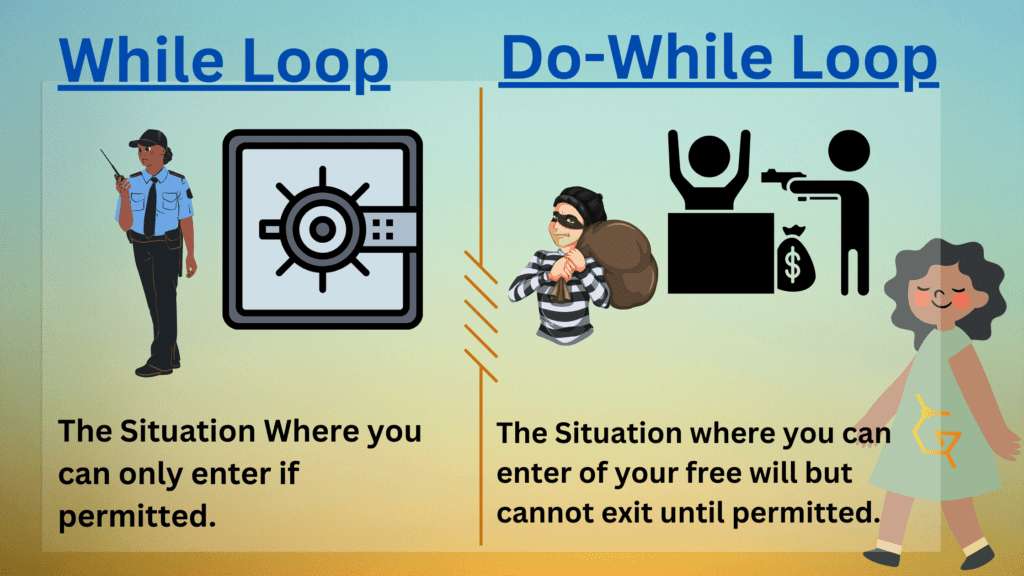
The Program
Objective
Write a Program using Do-While Loop to calculate the factorial of the number provided by the user.
Note: Factorials are large values to store, so here, we’re considering the factorial input as a non-zero value until 20 to work give the correct value.
The Code
#include <iostream>
using namespace std;
int main(){
//Part 1: Variable Initialization & User Input
int num;
long int fact=1;
cout<<"Enter Number: ";
cin>>num;
//Part 2: The main Looping Game
int i=num;
do{
fact*=i;
i--;
} while(i>=1);
//Part 3: The Output
cout<<"The Factorial of "<<num<<" is "<<fact;
return 0;
}
C++Output
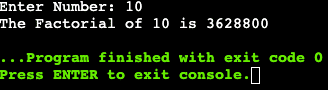
Explanation
In the program’s first part, we’ve initialized a long integer variable named fact with the value of 1, which will store the factorial. As factorials can be really big to store, normal integers could be small enough to store the bigger values.
Then we simply initialized a num variable and stored user input with valid choices in it.
//Part 1: Variable Initialization & User Input
int num;
long int fact=1;
cout<<"Enter Number: ";
cin>>num;
C++The second and main part is all about the calculations:
- Here we’re initializing the iterator and assigning the value of num to the iterator. As we know, factorial runs like 5! = 5*4*3*2*1 (* means multiply). Here we’re also trying to achieve the same by assigning the user input’s number to the iterator and decreasing it until 1 while multiplying it with the fact variable to store the factorial.
- Then we used the do-while loop with the condition to run the loop until the iterator is greater than equals 1 (i.e., including 1). That means the loop body has already executed once before checking the condition and deciding program flow.
- Inside the do block or loop body, the fact is multiplied by the iterator, and the iterator decreases until it reaches 1.
//Part 2: The main Looping Game
int i=num;
do{
fact*=i;
i--;
} while(i>=1);
C++In the third and last part, the print statement is used to provide the output to the user, simple 🙂
//Part 3: The Output
cout<<"The Factorial of "<<num<<" is "<<fact;
C++Conclusion
In this blog, we’ve covered the basic looping concepts of almost all major programming languages, which will enhance your coding skills to a great extent. By going through GeekonPeak’s detailed blog structure, you can also ensure that you’ll be easily able to counter the high-level interview questions based on these concepts. GeekonPeak has different and much more detailed explanations than ever; it’s the X-factor programmers exactly are looking for.
In the upcoming blogs, you’ll get exposure to continue statements and break statements. Also, check out previous tutorials, including for loop, while loop, and if-else block of statements, even the concept of the infinite time loop. This will complete the entire tutorial series course for C++ beginners to intermediate. After the C++ beginners to intermediate, the geeks plan to expand the peak with more extensive tutorials to enhance the learning base and provide more exclusive and practical learning.
This is also one of the most detailed blogs ever, and it took a lot of money & effort to upgrade the blog’s quality to this level. We’ve just begun. We’ve constantly improved the quality to enhance your experience, which shall require your support, and we expect it from you.
We shall start accepting donations soon as we’re spending heavily on the website’s maintenance and super high-quality content creation & research of the website. You can now support us through the like button on the bottom, sharing this blog, disabling ad blockers, commenting on your thoughts, and connecting with us on socials.
You can also support us by visiting this blog and buying the reviewed services (They’re tested and the best in the market), which will help us grow, develop, and upgrade the content quality to the next level. You may also subscribe to our newsletters, check out our latest blogs from other niches, and hit a like (heart) below the blog. Perfect Goodbye for now, and enjoy the ever-best programming blog series only at GeekonPeak!